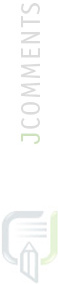
Developer's guide |
IntroductionJComments — component that can be easily integrated with any 3rd party extensions without any efforts. You must just add several code lines to your component and write simple plugin for JComments. The component utilizes plugins to obtain the information about commenting object (the object name and direct link). Plugins are necessary as the component has no information about commenting objects and data structure. That is why such tasks as object link creation and obtaining of its name are assigned to the extension developer. JComments plugin creationThe JComments plugin is a common php file that contains successor class of the base class for all plugins of JCommentsPlugin. This plugin contains two methods which are defined as:
The name of the exact class must contain two parts, the first with prefix jc_ and the second with component name for which the plugin was written. For example, if the plugin was written for the component com_mycomp the class must have the name: jc_com_mycomp. The name of the plugin file must contain also two parts: the first one with the component name and the second part of the file name is fixed ??? .plugin.php. For example, if the plugin was written for component com_mycomp, the file name of plugin must be: com_mycomp.plugin.php. After the plugin was created it must be placed in JComments plugin directory. It is planned to write the plugin installer, but in current version only manual installation is possible, i.e. it is necessary to copy the plugin file to folder /components/com_jcomments/plugins/ by FTP or some other way. Very simple plugin (other examples you can find in plugins directory): <?php class jc_com_mycomp extends JCommentsPlugin { function getObjectTitle( $id ) { // Data load from database by given id $db = & JFactory::getDBO(); $db->setQuery( "SELECT title FROM #__mycomp WHERE id='$id'"); return $db->loadResult(); } function getObjectLink( $id ) { // Itemid meaning of our component $_Itemid = JCommentsPlugin::getItemid( 'com_mycomp' ); // url link creation for given object by id $link = JRoute::_( 'index.php?option=com_mycomp&task=view&id='. $id .'&Itemid='. $_Itemid ); return $link; } function getObjectOwner( $id ) { $db = & JFactory::getDBO(); $db->setQuery( 'SELECT created_by, id FROM #__mycomp WHERE id = ' . $id ); return $db->loadResult(); } } ?> How to display the commentsYou have to include the main JComments file to display the comments somewhere and call the static method JComments::showComments. This method uses three parameters: object's ID, component name and object's title. $comments = JPATH_SITE . DS .'components' . DS . 'com_jcomments' . DS . 'jcomments.php'; if (file_exists($comments)) { require_once($comments); echo JComments::showComments($id, 'com_mycomp', $title); } Where $id— is commented object ID, com_mycomp - component name and $title— is the title of the current object. You can find the examples of usage of this method in ???Manual of JComments integration to third-party components???. How to display the comments quantityIf you would like to display somewhere the quantity of comments for any object (for example, to display the quantity on an object's intro page), you must include the main JComments file and call static method JComments::getCommentsCount through the function include/require. This method uses two parameters: object's ID and component name. $comments = JPATH_SITE . DS .'components' . DS . 'com_jcomments' . DS . 'jcomments.php'; if (file_exists($comments)) { require_once($comments); $count = JComments::getCommentsCount($id, 'com_mycomp'); echo $count ? ('Comments('. $count . ')') : 'Add comment'; } where $id — is the commented object ID and com_mycomp — component name. This code displays the 'Comments (5)' if you have 5 comments of given object and 'Add comments' if there are no comments. How to display the last comment of the objectIf you would like to display the last comment of any object, you must include the main JComments file through include/require and call static method JComments::getLastComment. This method uses two parameters: object's ID and component name. $comments = JPATH_SITE . DS .'components' . DS . 'com_jcomments' . DS . 'jcomments.php'; if (file_exists($comments)) { require_once($comments); $comment = JComments::getLastComment($id, 'com_mycomp'); echo 'User "' . $comment->name . '" wrote "' . $comment->comment . '" (' . $comment->date . ')'; } where the $id &mdash is the commented object ID and com_mycomp - component name. This code displays 'User "Administrator" wrote "This is sample comment" at "2007-02-07 16:52:53". How to delete all comments of the objectIf you would like to delete all comments of any object, you must include the main JComments file through include/require and call static method JComments::deleteComments. This method uses two parameters: object's ID and component name. $comments = JPATH_SITE . DS .'components' . DS . 'com_jcomments' . DS . 'jcomments.php'; if (file_exists($comments)) { require_once($comments); JComments::deleteComments($id, 'com_mycomp'); } where $id — is the commented object ID and com_mycomp — component name. After the method is called all comments of a given object will be deleted. How to delete all comments of the componentIf you would like to delete all comments of any object, you have to include the main JComments file through include/require and call static method JComments::deleteAllComments. This method uses only one parameter: component name. $comments = JPATH_SITE . DS .'components' . DS . 'com_jcomments' . DS . 'jcomments.php'; if (file_exists($comments)) { require_once($comments); JComments::deleteAllComments( 'com_mycomp' ); } After the method is called all comments of a given component will be deleted. |